19 Feb 2023
C Basics (Day 02_2)
C Basics
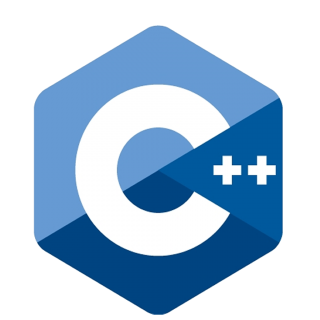
Keypoints
- Variables, assignments, data types
- collecting input
- arithmetic operators and precedence
Printing in C
// hello_world.c
#include <stdio.h>
// Print "Hello, world!" followed by newline and exit
int main(void){
printf("Helllo, world\n");
return 0;
}
// Compilation command line
$ gcc hello_world.c -std=c99 -pedantic -Wall -Wextra
$ ./a.out
// Output
Hello, world!
- printf allows for formatted printing of values, using placeholders in the format string
- eg:
printf("There are %d students in class.", 36);
- placeholders begin with ‘%’ and then contain additional format information regarding field size and precision, and lastly contains a character indicating the type of data to be inserted.
- the actual values corresponding to place holders are listed after the format string, 36 in this case.
- some of the most common data type place holders:
- d: decimal (integer type, ld for long int)
- u: unsigned (integer type that disallows negatives, lu for long unsigned)
- f: floating point (float, lf for double)
- c: character
- s: string
Variables
- When declared, a variable gets a type (int) and name (num_students)
- A variable also has a value that may change throughout the program’s lifetime
- To print the value:
printf("There are %d students in class.", num_students);
- Variable Type
- Integer types:
- int: signed integer, usually stored in 32 bits.
- unsigned: unsigned integer (positive integer).
- long: signed integer with much greater capacity than a plain int.
- Floating-point (decimal) types:
- float: single-precision floating point number.
- double: double-precision floating point number.
- Character type:
- char: holds a 1-byte character, ‘A’, ‘B’, ‘$’, etc.
- chars are basically integers.
- Boolean type:
- #include to use this
- bool: value can be true or false
- Integer values can also function as bools, where 0 means false, non-0 means true
Assignment
- = is the assignment operator, which modifies a variable’s value
- It’s strongly recommend to declare and assign at the same time:
- Generally, a variable declared but not assigned has an undefined value. Such undefined value should be avoided. It may cause fails might change from run to run. Undefined should strike fear into your heart.
Operators
- Addition: +
- Subtraction: -
- Multiplication: *
- Division: /
- Integer division: 7/2 = 3, not 3.5
- Remainder: %
Operator precedence
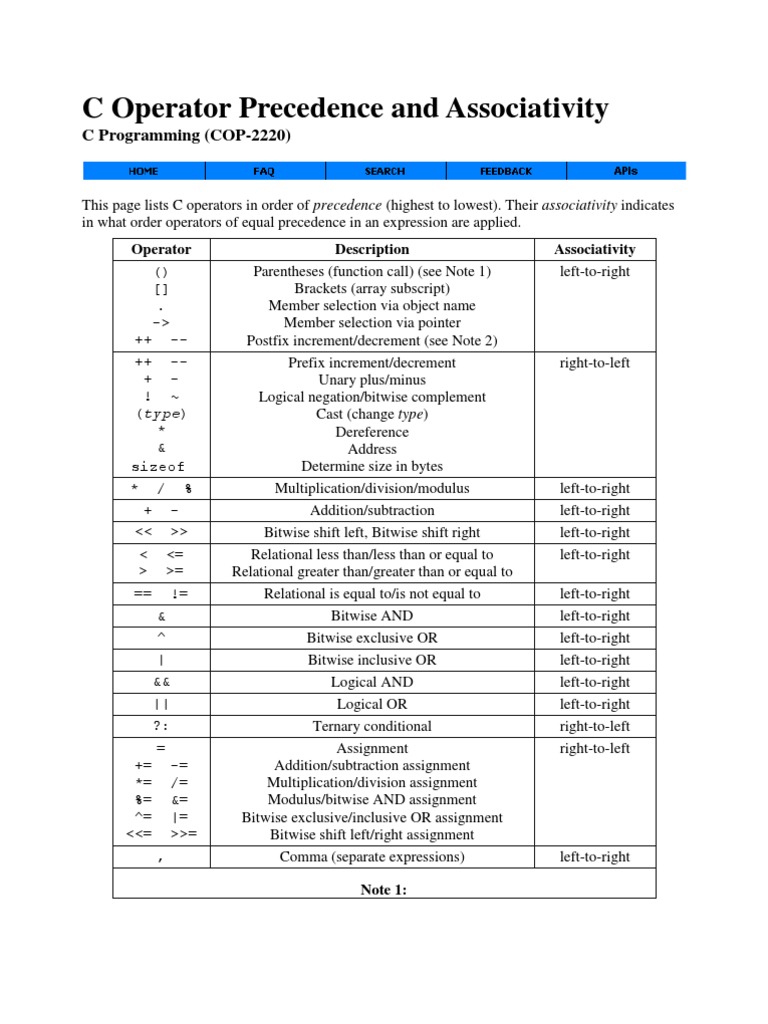
NOTE:
Using const
- Put cosnt before the type to say a variable cannot be modified
- The scanf function works similarly to the printf output function for reading formatted input: use a format string followed by the memory location we’re reading into.
//scanf_d.c:
int i;
printf("Please enter an integer: ");
scanf("%d", &i);
printf("The value you entered is %d", i);
- The memory location you indicate should be able to accommodate this type.
- The value scanf returns is the number of input items assigned
- 0: means the input is invalid for the specified type, even if input is available.
- EOF (which is -1): means no input at all was available. (end of file)