30 Apr 2023
C ++ Program Memory Division
C++ Basics
C ++ program memory division
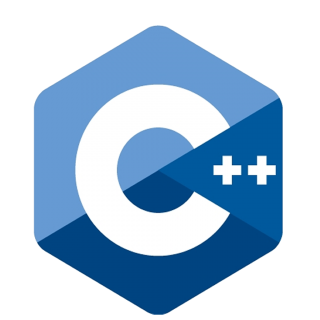
Keypoints
- Memory division classfication
Memory division classfication

- Significance: to bring different life cycle for different division, for dexterous programming.
Text/Code division
- Store the binary code of the correspondence, managed by the operating system. Assigned before program execution.
- Shared: for frequently run code, it’s sufficient to store only once.
- Only readable: not changable.
Global division
- Store global variables and static variables and constants. Assigned before program execution.
- Global variable: out of main function.
- Static variable: its address is near global ones.
static data_type variable_name = 10;
- Constant: string variable; const global variable. (constant local variable isn’t in Global division).
Stack division
- Automatically by compiler, to store function parameter and local variable.
- Don’t return address of local variable. Because stack data will be released after program execution.
int * func(){
int a = 10;
return &a;
}
int main(){
int *p = func();
cout << *p << endl; // return 10
cout << *p << endl; // return mess
cout << *p << endl; // return mess; because compiler store stack data only once, then it'll be released
}
Heap division
- Assigned and released by programmer. When program is over, OS will release it.
- Use new to start new heap area.
int * func(){
int *p = new int(10); // pointer here is a local variable and in stack area;
//new int (10) is not a value but an address
return p; // the data this pointer refers to is in heap area
}
int main(){
int *p = func(); // right, because 10 is in heap area
cout << *p << endl;
cout << *p << endl;
cout << *p << endl; // because programmer decide the release or not of heap data. It'll not be released automatically by compiler.
}
- Use new to start array in heap area. Use delete to release heap area.
int * func(){
int *p = new int(10); //new int (10) is not a value but an address/pointer
return p;
}
void test01(){
int *p = func();
cout << *p << endl;
cout << *p << endl;
cout << *p << endl;
delete p; // release heap area, if try to reach p again, it'll report error
}
void test02(){
int *arr = new int[10]; // [10] is array length
for (int i = 0; i < 10; i++){
arr[i] = i + 100;
}
for (int i = 0; i < 10; i++){
cout << arr[i] << endl;
}
delete[] arr; // release heap area
}
int main(){
test01();
test02();
}